Nested Loops
Matlab allows us to put compound statements like if
,
while
, and for
statements inside
other compound statements. This is called nesting.
You have already seen this capability using conditional (if
) statements.
Often, we must conditionally execute code (use if
stmts) within iterative
statements (loops). In this event, we nest (put) an if
statement in the body of the loop.
This nesting process can be done as many times as necessary.
Similarly, for
loops can be nested inside other for
loops,
or inside of while
and vice versa.
% create a multiplication table % and save it as A rows = 1:10 cols = 1:10 for r = rows for c = cols A(r, c) = r * c; end end
Keep in mind that just as with any statement that is put inside a for
loop,
a nested for
loop is executed once for every value in the vector of the
enclosing for
loop. In the following script, the outer for
loop
(the loop for r = rows) is executed 10 times, once for each value of r
.
Each time, the inner for
loop (the loop for c = cols)
updates 10 different entries, one for each value of c
,
in the A
matrix.
Therefore, a total of 100 (= 10 * 10) entries in A
are updated.
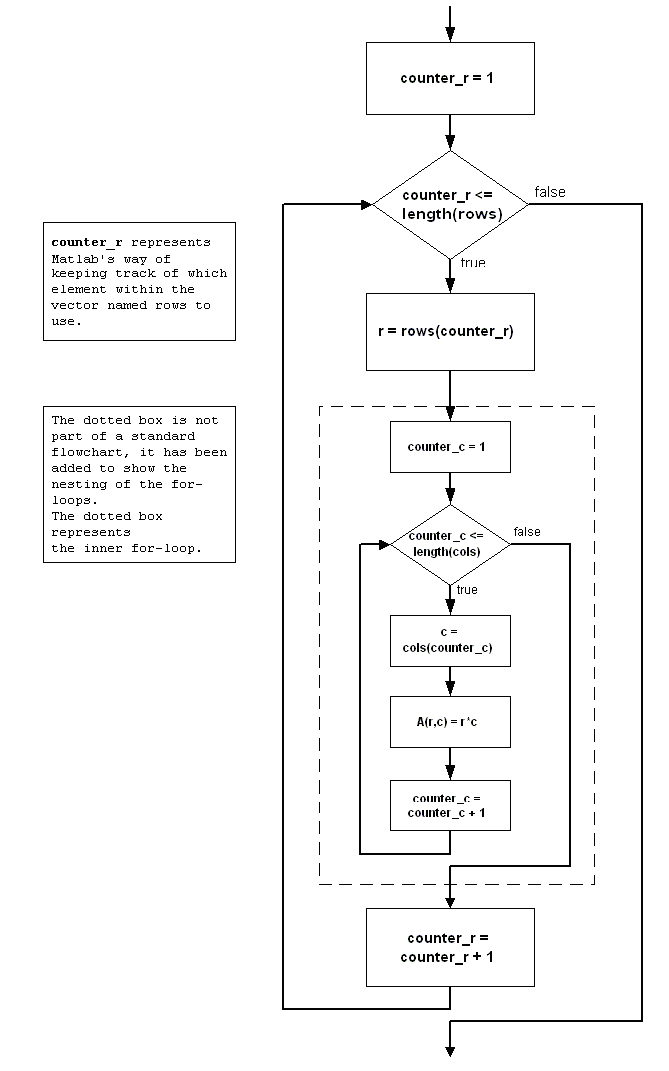
Indentation
When nesting if
statements and for
loops together,
consistent use of indentation helps to make your code readable.
Use the Smart Indent (Text->Smart Indent) feature of the Matlab
text editor to ensure that the indentation is correct for your code.
Improper indentation can hide problems:
Stopping a nested Loop Using the break Statement
Keep in mind that a break
statement only makes sense within a loop.
It is also important to understand that a break
statement only applies
to the inner-most loop enclosing the break statement.